new DialogHelper(params)
A helper class for adding studies to charts, modifying studies, and creating study edit dialog boxes.
Study DialogHelpers are created from study definitions or study descriptors (see the examples below).
A DialogHelper contains the inputs, outputs, and parameters of a study. Inputs configure the study. Outputs style the lines and filled areas of the study. Parameters set chart‑related aspects of the study, such as the panel that contains the study or whether the study is an underlay.
For example, a DialogHelper for the Anchored VWAP study with the axisSelect
parameter set to true
contains the following data:
inputs: Array(8)
0: {name: "Field", heading: "Field", value: "Close", defaultInput: "Close", type: "select", …}
1: {name: "Anchor Date", heading: "Anchor Date", value: "", defaultInput: "", type: "date"}
2: {name: "Anchor Time", heading: "Anchor Time", value: "", defaultInput: "", type: "time"}
3: {name: "Display 1 Standard Deviation (1σ)", heading: "Display 1 Standard Deviation (1σ)", value: false,
defaultInput: false, type: "checkbox"}
4: {name: "Display 2 Standard Deviation (2σ)", heading: "Display 2 Standard Deviation (2σ)", value: false,
defaultInput: false, type: "checkbox"}
5: {name: "Display 3 Standard Deviation (3σ)", heading: "Display 3 Standard Deviation (3σ)", value: false,
defaultInput: false, type: "checkbox"}
6: {name: "Shading", heading: "Shading", value: false, defaultInput: false, type: "checkbox"}
7: {name: "Anchor Selector", heading: "Anchor Selector", value: true, defaultInput: true, type: "checkbox"}
outputs: Array(4)
0: {name: "VWAP", heading: "VWAP", defaultOutput: "#FF0000", color: "#FF0000"}
1: {name: "1 Standard Deviation (1σ)", heading: "1 Standard Deviation (1σ)", defaultOutput: "#e1e1e1", color: "#e1e1e1"}
2: {name: "2 Standard Deviation (2σ)", heading: "2 Standard Deviation (2σ)", defaultOutput: "#85c99e", color: "#85c99e"}
3: {name: "3 Standard Deviation (3σ)", heading: "3 Standard Deviation (3σ)", defaultOutput: "#fff69e", color: "#fff69e"}
parameters: Array(4)
0: {name: "panelName", heading: "Panel", defaultValue: "Auto", value: "Auto", options: {…}, …}
1: {name: "underlay", heading: "Show as Underlay", defaultValue: false, value: undefined, type: "checkbox"}
2: {name: "yaxisDisplay", heading: "Y-Axis", defaultValue: "default", value: "shared", options: {…}, …}
3: {name: "flipped", heading: "Invert Y-Axis", defaultValue: false, value: false, type: "checkbox"}
which corresponds to the fields of the Study Edit dialog box:
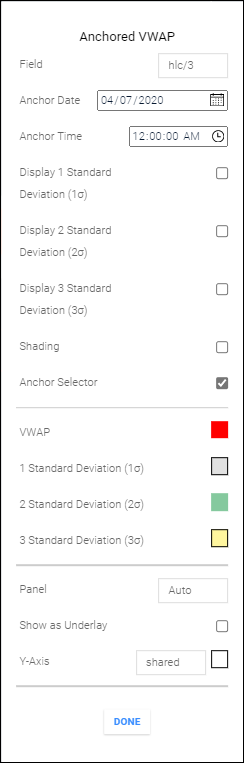
DialogHelpers also contain attributes
which specify the formatting of dialog box input
fields. For example, the DialogHelper for the Anchored VWAP study contains the following:
attributes:
Anchor Date: {placeholder: "yyyy-mm-dd"}
Anchor Time: {placeholder: "hh:mm:ss", step: 1}
The placeholder
property (in addition to its normal HTML function of providing placeholder
text) determines the input type of date and time fields. If the property value is "yyyy-mm-dd"
for a date field, the field in the edit dialog box is a date input type instead of a string
input. If the value is "hh:mm:ss" for a time field, the field is a time input type instead of a
string. If the hidden
property of a field is set to true, the field is excluded from the
study edit dialog box.
In the Anchored VWAP edit dialog box (see above), the Anchor Date field is formatted as a date input type; Anchor Time, as a time input type.
Note: Actual date/time displays are browser dependent. The time is displayed in the
displayZone
time zone. Time values are converted to the dataZone
time zone before being
used internally so they always match the time zone of masterData
. See
CIQ.ChartEngine#setTimeZone.
For more information on DialogHelpers, see the Using and Customizing Studies - Advanced tutorial.
Parameters:
Name | Type | Description | ||||||||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
params |
object | Constructor parameters. Properties
|
- Since:
-
- 6.3.0 Added parameters
axisSelect
andpanelSelect
. If a placeholder attribute ofyyyy-mm-dd
orhh:mm:ss
is set on an input field, the dialog displays a date or time input type instead of a string input type. - 7.1.0 It is expected that the study dialog's parameters section is refreshed whenever the DialogHelper changes. The "signal" member should be observed to see if it has flipped.
- 8.2.0 Attribute property values in the study definition can now be functions. See the Input Validation section of the Using and Customizing Studies - Advanced tutorial.
- 6.3.0 Added parameters
- See:
-
- CIQ.Studies.addStudy to add a study to the chart using the inputs, outputs, and parameters of a DialogHelper.
- CIQ.Studies.DialogHelper#updateStudy to add or modify a study.
- CIQ.UI.StudyEdit to create a study edit dialog box using a DialogHelper.
Examples
Create a DialogHelper from a study definition.
let helper = new CIQ.Studies.DialogHelper({ name: "ma", stx: stxx })
Create a DialogHelper from a study descriptor.
let sd = CIQ.Studies.addStudy(stxx, "Aroon");
let helper = new CIQ.Studies.DialogHelper({ sd: sd, stx: stxx, axisSelect: true });
Display the DialogHelper inputs, outputs, parameters, and attributes.
let helper = new CIQ.Studies.DialogHelper({ name: "stochastics", stx: stxx });
console.log("Inputs:", JSON.stringify(helper.inputs));
console.log("Outputs:", JSON.stringify(helper.outputs));
console.log("Parameters:", JSON.stringify(helper.parameters));
console.log("Attributes:", JSON.stringify(helper.attributes));
Methods
-
adjustInputTimesForDisplayZone( [updates])
-
Adjust all date and time fields in the DialogHelper to use the display zone.
This function can adjust both to and from the display zone depending on the presence of the second argument. When creating the DialogHelper, the second argument is null, and any date and time in the study descriptor's inputs is converted to display zone when stored in the DialogHelper's
inputs
property. When updating the DialogHelper, the second argument contains any changed fields. If a date or time has been changed, it is converted back from display zone so it can be stored correctly in the study descriptor. It is assumed that the updated date and time are in display zone already. The function adjusts the time by changing theupdates
object if it is passed, or theinputs
property if it is not.In the example below, it is assumed that there are input fields named "Anchor Date" and "Anchor Time". Whenever you want to set up an input field with date and time, use this convention: Name both fields the same name and add " Date" to one and " Time" to the other.
Parameters:
Name Type Argument Description updates
Object <optional>
If updating, it should contain an object with updates to the
inputs
object used in CIQ.Studies.addStudy.- Since:
-
6.3.0
Example
var helper=new CIQ.Studies.DialogHelper({sd:sd, stx:stx}); var updates={inputs:{"Anchor Time":"06:00"}}; helper.adjustInputTimesForDisplayZone(updates});
-
updateStudy(updates)
-
Updates or adds the study represented by the DialogHelper.
When a study has been added using this function, a study descriptor is stored in the
sd
property of the DialogHelper.When a study has been updated using this function, all DialogHelper properties, including
sd
, are updated to reflect the changes. However, other DialogHelper instances of the same study type are not updated. For example, the inputs, outputs, and parameters of a DialogHelper will not contain any new values as a result of another DialogHelper's update.Parameters:
Name Type Description updates
object Contains values for the
inputs
,outputs
, andparameters
properties of the DialogHelper.- Since:
-
6.3.0 This DialogHelper instance is refreshed after an update; recreating it is no longer necessary.
Examples
Add and update a study.
// Add the study. let aroonSd = CIQ.Studies.addStudy(stxx, "Aroon"); // Create a DialogHelper. let dialogHelper = new CIQ.Studies.DialogHelper({ stx: stxx, sd: aroonSd }); // Move the study to the chart panel. dialogHelper.updateStudy({ parameters: { panelName: "chart" } }); // Move the study back to its own panel. dialogHelper.updateStudy({ parameters: { panelName: "New panel" } });
Add a customized study.
let helper = new CIQ.Studies.DialogHelper({ stx: stxx, name: "AVWAP" }); helper.updateStudy({ inputs: { Field: "High" }, outputs: { VWAP: "#ff0" }, parameters: { panelName: "New Panel" } });
Update a study and get the updated study descriptor.
let helper = new CIQ.Studies.DialogHelper({ stx: stxx, name: "Aroon" }); helper.updateStudy({ inputs: { Period: 60 } }); let updatedSd = helper.sd;